For part 2, I will be sharing the result using the x86_64 platform. I will be using the servers that are provided to us to test this lab. In this lab, we will be looping "Hello World" as well as counting the loop with a max number of 30.
For more detail information of this lab, you can access this link (https://wiki.cdot.senecacollege.ca/wiki/SPO600_64-bit_Assembler_Lab)
For part 2 of lab 5, we will be working in x86_64 assembler. This code for the loop was provided to us in class:
.text .globl _start min = 0 /* starting value for the loop index; note that this is a symbol (constant), not a variable */ max = 10 /* loop exits when the index hits this number (loop condition is i<max) */ _start: mov $min,%r15 /* loop index */ loop: /* ... body of the loop ... do something useful here ... */ inc %r15 /* increment index */ cmp $max,%r15 /* see if we're done */ jne loop /* loop if we're not */ mov $0,%rdi /* exit status */ mov $60,%rax /* syscall sys_exit */ syscall
First, we build and run three C versions of the program for x86_64 and then proceed to use objdump -d hello and this is what we got for the <main> portion:
hello
0000000000401126 <main>:
401126: 55 push %rbp
401127: 48 89 e5 mov %rsp,%rbp
40112a: bf 10 20 40 00 mov $0x402010,%edi
40112f: b8 00 00 00 00 mov $0x0,%eax
401134: e8 f7 fe ff ff callq 401030 <printf@plt>
401139: b8 00 00 00 00 mov $0x0,%eax
40113e: 5d pop %rbp
40113f: c3 retq
hello2
0000000000401126 <main>:
401126: 55 push %rbp
401127: 48 89 e5 mov %rsp,%rbp
40112a: ba 0d 00 00 00 mov $0xd,%edx
40112f: be 10 20 40 00 mov $0x402010,%esi
401134: bf 01 00 00 00 mov $0x1,%edi
401139: e8 f2 fe ff ff callq 401030 <write@plt>
40113e: b8 00 00 00 00 mov $0x0,%eax
401143: 5d pop %rbp
401144: c3 retq
401145: 66 2e 0f 1f 84 00 00 nopw %cs:0x0(%rax,%rax,1)
40114c: 00 00 00
40114f: 90 nop
hello3
0000000000401126 <main>:
401126: 55 push %rbp
401127: 48 89 e5 mov %rsp,%rbp
40112a: b9 0d 00 00 00 mov $0xd,%ecx
40112f: ba 10 20 40 00 mov $0x402010,%edx
401134: be 01 00 00 00 mov $0x1,%esi
401139: bf 01 00 00 00 mov $0x1,%edi
40113e: b8 00 00 00 00 mov $0x0,%eax
401143: e8 e8 fe ff ff callq 401030 <syscall@plt>
401148: b8 00 00 00 00 mov $0x0,%eax
40114d: 5d pop %rbp
40114e: c3 retq
40114f: 90 nop
Note:
We can see that hello2 and hello3 are very similar in their <main> but hello <main> have fewer lines than both of them.
Code provided to us as a starting point:
/*
This is a 'hello world' program in x86_64 assembler using the
GNU assembler (gas) syntax. Note that this program runs in 64-bit
mode.
CTyler, Seneca College, 2014-01-20
Licensed under GNU GPL v2+
*/
.text
.globl _start
_start:
movq $len,%rdx /* message length */
movq $msg,%rsi /* message location */
movq $1,%rdi /* file descriptor stdout */
movq $1,%rax /* syscall sys_write */
syscall
movq $0,%rdi /* exit status */
movq $60,%rax /* syscall sys_exit */
syscall
.section .rodata
msg: .ascii "Hello, world!\n"
len = . - msg
The second part of this lab is to Loop 0-30, results:
.text
.globl _start
_start:
movq $min, %r10 /*stores the min vale into %r10*/
movq $division, %r9 /*stores the division value 10 into %r9*/
loop:
cmp %r9, %r10 /*compare %r9 with %r10*/
jl digit_1 /* if the value is less or equal than 9(1-digit), go to the subroutine digit_1*/
jmp digit_2/* if the value is greater than 9(2-digit), go to the subroutine digit_2*/
digit_1:
movq %r10, %r15
add $'0', %r15 /*ascii number character*/
movq $msg+15, %r11 /* digit location within string*/
movb %r15b, (%r11) /*store the digit at the location */
jmp print /*go to print subroutine*/
digit_2:
movq $0, %rdx
movq %r10, %rax
div %r9
movq %rax, %r14
movq %rdx, %r15
add $'0', %r14/*ascii number character*/
add $'0', %r15/*ascii number character*/
movq $msg+14, %r11/* digit location within string*/
movb %r14b, (%r11) /*store the digit at the location */
movq $msg+15, %r12/* digit location within string*/
movb %r15b, (%r12) /*store the digit at the location */
jmp print/*go to print subroutine*/
print:
movq $len,%rdx /* message length */
movq $msg,%rsi /* message location */
movq $1,%rdi /* file descriptor stdout */
movq $1,%rax /* syscall sys_write */
syscall
inc %r12b
inc %r10
cmp $max, %r10
jne loop
movq $0,%rdi /* exit status */
movq $60,%rax /* syscall sys_exit */
syscall
.section .data
msg: .ascii "Hello, world!: 0\n"
len = . - msg
min = 0
max = 30
division = 10
Output:
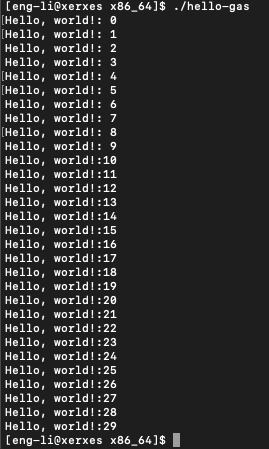
This part of the lab was kinda easy because we already implemented this code on the Aarch64, so what we did is change the wording into x86_64, we did encounter some problems such as the loop wasn't working that well and then we realize that we used the incorrect register or where we store our digits were in the incorrect register.
Thoughts:
This part of the lab was really fun to do since we just experiment with x86_64, I will say both x86_64 and Aarch64 are relatively similar but both of them have their own issues. I don't have a preference yet with both of these assembly languages, I would say that I prefer both of them than 6502.
Maybe with the future labs, I will change my opinion on both of these servers and might pick which one I like better. But, so far I do not have a favourite yet.
Comments