This lab will be exploring the different servers such as x86_64 and AArch64. For both servers, we will be doing a simple loop of "Hello World" as well as counting them with max number 30.
For more detail information of this lab, you can access this link (https://wiki.cdot.senecacollege.ca/wiki/SPO600_64-bit_Assembler_Lab)
For part 1 of lab 5, we will we work first in AArch64 assembler. We used this code for loop control that was provided for us in class:
.text .globl _start min = 0 /* starting value for the loop index; note that this is a symbol (constant), not a variable */ max = 30 /* loop exits when the index hits this number (loop condition is i<max) */ _start: mov x19, min loop: /* ... body of the loop ... do something useful here ... */ add x19, x19, 1 cmp x19, max b.ne loop mov x0, 0 /* status -> 0 */ mov x8, 93 /* exit is syscall #93 */ svc 0 /* invoke syscall */
First, we build and run three C versions of the program for AArch64 and then proceed to use objdump -d hello and this is what we got for the <main> portion:
hello
000000000040059c <main>:
40059c: a9bf7bfd stp x29, x30, [sp, #-16]!
4005a0: 910003fd mov x29, sp
4005a4: 90000000 adrp x0, 400000 <_init-0x418>
4005a8: 9119e000 add x0, x0, #0x678
4005ac: 97ffffb5 bl 400480 <printf@plt>
4005b4: a8c17bfd ldp x29, x30, [sp], #16
4005b8: d65f03c0 ret
4005bc: d503201f nop
hello2
000000000040059c <main>:
40059c: a9bf7bfd stp x29, x30, [sp, #-16]!
4005a0: 910003fd mov x29, sp
4005a8: 90000000 adrp x0, 400000 <_init-0x418>
4005ac: 911a0001 add x1, x0, #0x680
4005b4: 97ffffaf bl 400470 <write@plt>
4005bc: a8c17bfd ldp x29, x30, [sp], #16
4005c0: d65f03c0 ret
4005c4: d503201f nop
hello3
000000000040059c <main>:
40059c: a9bf7bfd stp x29, x30, [sp, #-16]!
4005a0: 910003fd mov x29, sp
4005a8: 90000000 adrp x0, 400000 <_init-0x418>
4005ac: 911a0002 add x2, x0, #0x680
4005b8: 97ffffb2 bl 400480 <syscall@plt>
4005c0: a8c17bfd ldp x29, x30, [sp], #16
4005c4: d65f03c0 ret
Notes:
hello2 and hello3 are similar in their <main> but hello have fewer lines than both of them.
Second, as a starting point of our lab, this code was provided to us
.text
.globl _start
_start:
mov   x19,min
loop:
mov   x0, 1      /* file descriptor: 1 is stdout /
adr   x1, msg     / message location (memory address) /
mov   x2, len     / message length (bytes) /
mov   x8, 64     / write is syscall #64 /
svc   0        / invoke syscall /
add   x19, x19, 1
cmp   x19, max
b.ne  loop
mov   x0, 0      / status -> 0 /
mov   x8, 93     / exit is syscall #93 /
svc   0        / invoke syscall */
.data
msg:  .ascii   "Hello, world!\n"
len=Â Â . - msg
min = 0
max = 30
Note: We can see that this loop code is similar to the C language code:
for(int i = 0; i < 30, i++){
printf("Hello, world!\n");
}
Code used for the Loop 0-30:
.text
.globl _start
_start:
mov x19, min /*store the min value into x19*/
mov x18,division /*store the division value 10 into x18*/
loop:
mov x0, 1 /* file descriptor: 1 is stdout */
cmp x19, 9 /* compare x19(loop index value) with 9*/
b.gt digit_2 /* if the value is greater than 9(2-digit), go to the subroutine digit_2*/
bl digit_1 /* if the value is less or equal than 9(1-digit), go to the subroutine digit_1*/
digit_1:
add x20, x19, '0' /* ascii number character*/
adr x30, msg+14 /* the digit location within string */
strb w20, [x30] /* store the digit at the location */
bl print /* go to the print subroutine */
digit_2:
udiv x25, x19, x18 /* divide the value by 10 and store the value into the x25 */
msub x26, x25, x18, x19 /* store the remainder into x26 */
add x21, x25, '0' /* ascii number character */
add x20, x26, '0' /* ascii number character */
adr x25, msg+14 /* the digit location within string */
strb w21, [x25] /* store the digit at the location */
adr x26, msg+15 /* the digit location within string */
strb w20, [x26] /* store the digit at the location */
bl print
print:
adr x1, msg /* store the locatio of message */
mov x2, len /* store the string length into x2 */
mov x8, 64 /* write is syscall #64 */
svc 0 /* invoke syscall */
add x19, x19, 1 /* increment x19 value which is loop index */
cmp x19, max /* compare x19 with max value */
b.ne loop /* if the value is not equal to max value, loop it again */
mov x0, 0 /* status -> 0 */
mov x8, 93 /* exit is syscall #93 */
svc 0 /* invoke syscall */
.data
msg: .ascii "Hello World!: \n"
len= . - msg
min = 0 /* starting value for the loop index; note that this is a symbol (constant), not a variable */
max = 30 /* loop exits when the index hits this number (loop condition is i<max) */
division=10
Output:
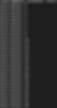
We had some problems while implementing this lab, such as Segmentation Fault. In the beginning, we were comparing using 'b.ne' and 'b.gt' while the correct way to this was 'bl'. Because we were trying to jump from a subroutine but did not know how to do it until we went back to our lectures note and realize our mistake.
Thoughts:
At the beginning of this lab, I had some problems trying to access the server but I got it to fix with the help of my group and instructor. Overall the experience of this lab was really good, I got to see the differences between 6502 and Aarch64 assembler. In my personal opinion, I rather work with Aarch64 or x86_64 than 6502 because I understand it more and I know what I am doing.
The second part of this Lab 5 is basically the same thing but we using x86_64 assembler. I will be posting the results soon once I fix the issues that I have with my server.